一、MAT是什么?
MAT(Memory Analyzer Tool),一个基于Eclipse的内存分析工具,是一个快速、功能丰富的JAVA heap分析工具,它可以帮助我们查找内存泄漏和减少内存消耗。使用内存分析工具从众多的对象中进行分析,快速的计算出在内存中对象的占用大小,看看是谁阻止了垃圾收集器的回收工作,并可以通过报表直观的查看到可能造成这种结果的对象。
二、如何使用
使用的方法将dump文件导入然后进行分析。
方法如下:
1、通过JMX的MBean生成当前的Heap信息,大小为一个3G(整个堆的大小)的hprof文件,如果没有启动JMX可以通过Java的JMAP命令来生成该文件。
2、 要考虑的是如何打开这个DUMP的堆信息文件,显然一般的Window系统没有这么大的内存,必须借助高配置的Linux。当然我们可以借助X-Window把Linux上的图形导入到Window。我们考虑用下面几种工具打开该文件:
Visual VM、IBM HeapAnalyzer、JDK 自带的Hprof工具。
使用这些工具时为了确保加载速度,建议设置最大内存为6G。使用后发现,这些工具都无法直观地观察到内存泄漏,Visual VM虽能观察到对象大小,但看不到调用堆栈;HeapAnalyzer虽然能看到调用堆栈,却无法正确打开一个3G的文件。可以使用MAT工具直接导入文件,生成图表信息和疑似有问题的JAVA类,如下图所示:
***************************原文结束,学习笔记开始***************************
之前的关于OOM异常的学习笔记提到过,对于出现内存泄露等疑难问题,需要保留日志,mat进行分析。
那就开搞吧,体会下过程。
1安装mat。
要是MyEclipse,网上有教程怎么安装,我本机安装的事Spring Tool Suite Version: 3.5.0.RELEASE.
也可以从sts - >help - > Install New Software。
https://www.eclipse.org/mat/downloads.php
这是下载页面。里面有对应的地址。copy出来。
在workwith输入。选择第一个,然后一路安装见下图。
又发现一个问题。jdk我安装的事
64位,但是sts是同事拷的32位的,所以下载的插件也是32位的,没法运行,悲剧了 没办法,直接下载MemoryAnalyzer-1.5.0.20150527-win32.win32.x86_64.zip。解压缩后直接运行。
****************安装完成,进入分析dump阶段****************** !
ENTRY org.eclipse.mat.ui 4 0 2016-03-09 14:41:27.773 !MESSAGE Error opening heap dump '31615.dump'. Check the error log for further details. org.eclipse.mat.SnapshotException: Error opening heap dump '31615.dump'. Check the error log for further details. at org.eclipse.mat.parser.internal.SnapshotFactoryImpl.parse(SnapshotFactoryImpl.java:268) at org.eclipse.mat.parser.internal.SnapshotFactoryImpl.openSnapshot(SnapshotFactoryImpl.java:126) at org.eclipse.mat.snapshot.SnapshotFactory.openSnapshot(SnapshotFactory.java:145) at org.eclipse.mat.ui.snapshot.ParseHeapDumpJob.run(ParseHeapDumpJob.java:83) at org.eclipse.core.internal.jobs.Worker.run(Worker.java:55) Caused by: java.io.IOException: Invalid HPROF file header. at org.eclipse.mat.hprof.AbstractParser.readVersion(AbstractParser.java:143) at org.eclipse.mat.hprof.Pass1Parser.read(Pass1Parser.java:84) at org.eclipse.mat.hprof.HprofIndexBuilder.fill(HprofIndexBuilder.java:80) at org.eclipse.mat.parser.internal.SnapshotFactoryImpl.parse(SnapshotFactoryImpl.java:222) 上网搜了下,Google有类似报错,我理解mat只能打开二进制文件。
还没测试完呢不能放弃,自己去生成一个吧。jmap ,这次注意了加了format=b
果然比较大,900多M。下载到本机。
-rw------- 1 cloud-user cloud-user 907M Mar 9 15:26 8423
打开刚才下载文件。“File->Open heap dump...”打开指定的dump文件后,将会生成Overview选项,
,当然对于我的烂电脑来说是个有点漫长的过程,内存快满了,期间很卡。如下图所示:
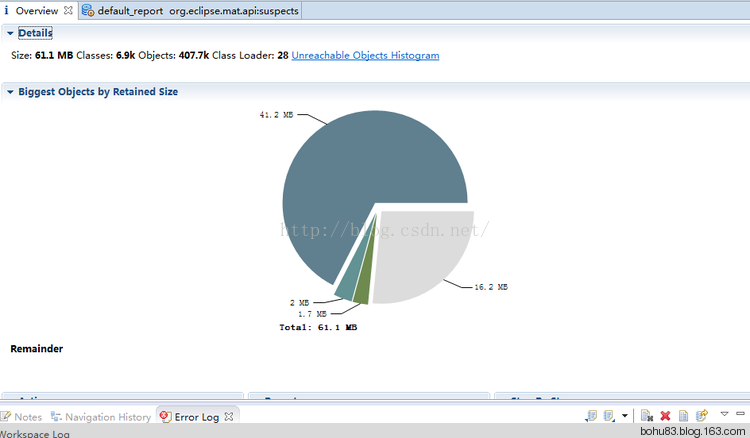
在Overview选项中,以饼状图的形式列举出了程序内存消耗的一些基本信息,其中每一种不同颜色的饼块都代表了不同比例的内存消耗情况。
1. Histogram可以列出内存中的对象,对象的个数以及大小。
2. Dominator Tree可以列出那个线程,以及线程下面的那些对象占用的空间。
3.Top consumers通过图形列出最大的object。
4.Leak Suspects通过MA自动分析泄漏的原因。
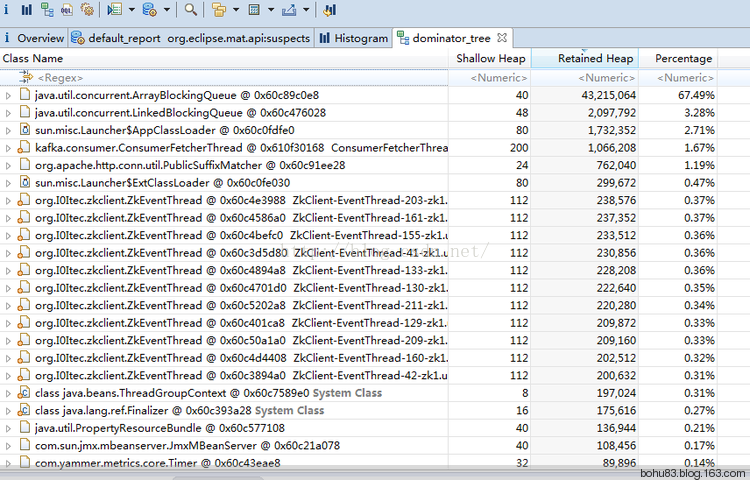
最后,再来看看 Leak Suspects
System Overview
One instance of "java.util.concurrent.ArrayBlockingQueue" loaded by "<system class loader>" occupies 43,215,064 (67.49%) bytes. The instance is referenced by com.hshbic.uplus.collect.Thread.QueueObject @ 0x60c89c0d8 , loaded by "sun.misc.Launcher$AppClassLoader @ 0x60c0fdfe0". The memory is accumulated in one instance of "java.lang.Object[]" loaded by "<system class loader>". Keywords java.util.concurrent.ArrayBlockingQueue java.lang.Object[] sun.misc.Launcher$AppClassLoader @ 0x60c0fdfe0
点击details,可以查看详情
我想在实际应用中,也是一问题为导向,如果看到频繁触发GC,就是借助工具看看是那些类占用内存大小,影响回收。